HTML Div Tag
The <div> tag is one of the most commonly used tags in HTML. It helps to divide a webpage into sections. It acts as a container for different content, which can be styled using CSS or controlled with JavaScript. You can easily customize it with class or id attributes, and it can hold various types of content like text, images, and links. This makes it useful for organizing web pages.
- Block-Level Element: The
<div>
tag is a block-level element, meaning it takes up the full width and starts on a new line. - Styling with CSS: It can be styled using CSS, allowing customization like width, background color, padding, and margins.
- Inline-Block Option: By using
display: inline-block
, a<div>
can behave like an inline element while retaining block-level features. - Used for Layouts:
<div>
is often used in layouts with Flexbox or Grid to organize content and arrange sections of a webpage.
Syntax
<div>
<!-- Content goes here -->
</div>
Now, let us understand with the help of the example:
<html>
<head>
<style>
/* Basic styling for better readability */
body {
font-family: Arial, sans-serif;
margin: 20px;
background-color: #f4f4f4;
}
/* Styling for the div blocks */
div {
color: white;
background-color: #009900;
/* Fixed the color */
margin: 10px 0;
padding: 10px;
font-size: 20px;
border-radius: 5px;
/* Optional: Adds rounded corners */
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
/* Optional: Adds subtle shadow */
}
/* Headings for clarity */
h1 {
font-size: 28px;
color: #333;
}
/* Styling for the section and article */
section {
margin-top: 30px;
}
/* Responsive styling */
@media (max-width: 600px) {
div {
font-size: 18px;
padding: 8px;
}
h1 {
font-size: 24px;
}
}
</style>
</head>
<body>
<article>
<h1>Understanding the <code>div</code> Tag in HTML</h1>
<section>
<p>The <code>div</code> tag is a container element used to group content together.
It's a commonly used tag for styling sections of a page.</p>
<div>First div tag with some content</div>
<div>Second div tag, providing another example</div>
<div>Third div tag demonstrating further usage</div>
<div>Fourth div tag showing how to style multiple blocks</div>
</section>
</article>
</body>
</html>
Output
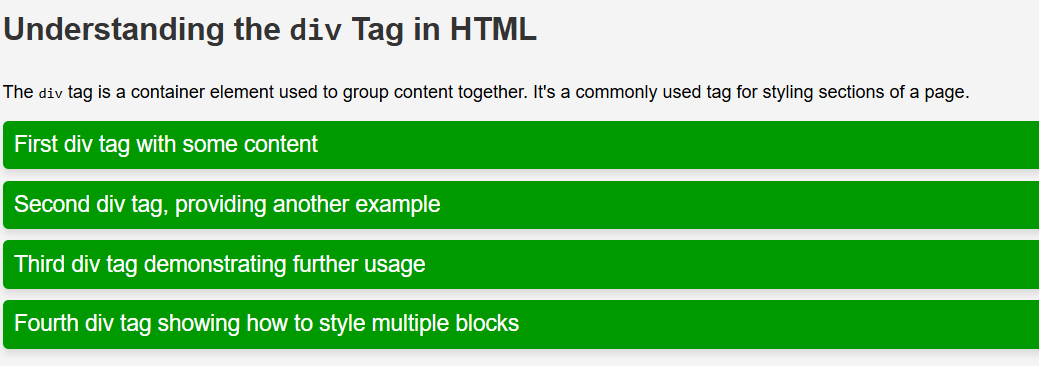
HTML Div Tag
In this example:
- Structure: The page has a title and content inside an article.
- Styling: The
<div>
elements are green with white text, padding, rounded corners, and shadow. - Div Elements: Four
<div>
blocks are used to group content. - Heading: The heading explains the
<div>
tag. - Responsive Design: The page adjusts for smaller screens with smaller text and padding.
How to Style the div Tag
The <div> tag is easy to style and is often used by developers to group content together. It accepts almost all CSS properties, making it very versatile. Here’s how to style a <div> tag in a simple way:
1. Apply Font Properties with div
The <div> tag can be styled using properties like font-size, font-family, font-weight, and font-style to control the appearance of text
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Font Properties Example</title>
<style>
.text-style {
font-family: 'Arial', sans-serif; /* Font type */
font-size: 24px; /* Font size */
font-weight: bold; /* Makes text bold */
}
</style>
</head>
<body>
<div class="text-style">
This is a simple example of applying font properties to a div element.
</div>
</body>
</html>
Output

HTML Div Tag
2. Apply Color with the Div Tag
The color and background-color properties change the text color and background color of the content inside the <div>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Font Properties Example</title>
<style>
.text-style {
font-family: 'Arial', sans-serif;
font-size: 24px;
font-weight: bold;
color: blue;
}
</style>
</head>
<body>
<div class="text-style">
This is a example of applying font properties to a div element.
</div>
</body>
</html>
Output

HTML Div Tag
3. Style Texts with the Div Tag
The text-transform and text-decoration properties can be used to change the style of text inside a <div>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Style Texts with the div Tag</title>
<style>
.text-style {
color: green;
text-align: center;
text-decoration: underline;
text-transform: uppercase;
font-size: 30px;
}
</style>
</head>
<body>
<div class="text-style">
This is a styled text inside a div element.
</div>
</body>
</html>
Output

HTML Div Tag
4. Create a Shadow Effect with the Div Tag
The box-shadow property adds a shadow effect to a <div>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Shadow Effect with div</title>
<style>
.shadow-effect {
width: 300px;
height: 200px;
background-color: lightblue;
margin: 50px auto;
box-shadow: 10px 10px 20px rgba(0, 0, 0, 0.3);
padding: 20px;
font-size: 20px;
text-align: center;
}
</style>
</head>
<body>
<div class="shadow-effect">
This div has a shadow effect.
</div>
</body>
</html>
Output
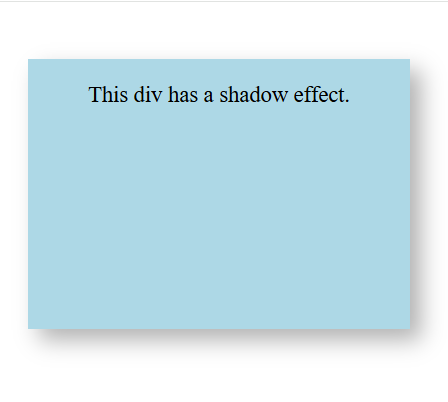
HTML Div Tag Shadow Effect
Best Practices for Using the <div>
Tag
To use the <div>
tag effectively, consider the following best practices:
- Use Semantic HTML Tags When Possible: While
<div>
is great for layout and grouping, try to use more descriptive, semantic tags like<header>
,<footer>
,<article>
,<section>
, and<aside>
for better accessibility and SEO. - Limit the Use of
<div>
Tags: Avoid overusing<div>
tags unnecessarily. Excessive use of<div>
elements can make your HTML code less readable and harder to maintain. Only use<div>
when other tags are not appropriate. - Use Classes and IDs for Styling: When styling
<div>
elements, use classes or IDs to target specific<div>
tags, making your styles more organized and reusable. - Keep the Structure Clean: Properly structure your
<div>
tags by nesting them logically and keeping your HTML as semantic as possible. This will improve maintainability and readability.
Conclusion
The <div>
tag is a fundamental part of web development, offering flexibility and versatility for grouping content and applying styles. While it’s a crucial element for structuring pages, it should be used wisely in combination with more semantic HTML tags and CSS for effective and maintainable web design.